Ruby Programming Language Tutorials
The language of these tutorials is very easy. In these tutorial you will learn ruby programming language very easily. Enjoy programming
hl
Friday, 5 October 2018
Wednesday, 5 April 2017
Ruby Programming Language Tutorials Lec 14
Tutorial 14 : Cont. to strings
Conversion of a string
If you want
to convert a string into integer so you can do is to write the name of the
string and then write dot operator and the write to_i
If you want
to convert a string into float values so you can do is to write the name of the
string and then write dot operator and the write to_f
If you want
to convert a string into symbols so you can do is to write the name of the
string and then write dot operator and the write to_sym
Examples :
Program in RUBY of objects
Okay everything
inside the ruby is an object and now we will talk about the objects. We are
going to model an object using classes like everyday objects. Every object is going
to have some attributes. Which we going to call instance variables as well as keep
abilities which we going to call methods. Now let’s say we have a class called
animal inside of it you can put an initialize function and this is called any
time new animal objects in this you create default values let’s say creating a
new animal and end it of course
I am going
to show you how to create setters and getters inside ruby but for now let’s
create setter and getter. Let’s set by pass new_name. An instance variable
inside the ruby by @ symbol and then name no need to do anything else and then
equals operator and then write the name of the variable such as new_name it
will assign the value to that. After that we going to put the end
And then we define a getter by typing def
keyword and then write get_name you just put @name it will return that. After
that end it. After that you command there
And another type you can make setter is to
just def name = (new_name). Let’s make sure that we are passing name which is
numeric. So make a condition of if the string is numeric write if new_name.is_a?
(Numeric) puts “Name Can’t Be a Number” something like that. Else and then we
going to assign the values that will passed in the name instance variable and
then we end the else. And then we end the setter method and finally we going to
end the class.
As we create a class we is to create an object. So let’s create a cat name object by typing cat = Animal.new and then we set the name peekaboo to the cat and then we make a getter here by cat.get_name after that we going to print to out by puts cat.name. Let me show you some short cut to set some getter and setter. Within a second write cat.name = “sophie”
And for
write puts cat.name
Output
Ruby Programming Language Tutorials Lec 13
Tutorial 13 : Cont. to strings
Use of CHOP and CHOMP
To remove the last charecter from the string we use chop by typing string_name and then dot operator and then write chop. your last character is chopped out like if you want only first name in the full name so write full_name.chop the it will remove the last part from the full name
And the CHOMP work same as chop works but it delete multiple letters
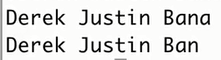

Input Output
Delete
There is a keyword in ruby to delete a
letter in the whole string. Such as if you want to delete a letter from the
string full name you is to type full_name.delete(“a”) it will do delete the
letter a from the string. So let’s check it out
Example is below with split
Split
To split every letter in the string first
write name of the string and then write dot operator and the write split it
will do split the string but it is not full for every letter pass // in the parenthesis.
For split the words do the same but pass
the / /with space between them by which it will split the words out
Input Output
NOTE: It
will also count white spaces as a letter
Thursday, 30 March 2017
Ruby Programming Language Tutorials Lec 12
Tutorial 12 : Cont. to strings
Equality in Strings
For equality in strings we are to simply write in double quote and we write a string and then write . operator and after that write equal and the put a question mark there and then write the equal string which you want to be equal in double quote. Let's check it out
Input Output
Input Output
Input Output
And if we write first_name.equal?first_name it will give result true because they are the exactly same objects.
Changing uppercase Lowercase and Swapcase
To change a string in uppercase type the name of the string and write .upcase it will convert the whole string to the uppercase. Similarly for the lowercase and for the swapcase write downcase and swapcase. So let's check it out
Input Output
Manipulation With Spaces in a String
If we added some extra spaces on the left side of a string or the right side of a string and it is taking some extra space in our memory so here we can swipe the white spaces by typing string name.lstrip for left removal of the white spaces .rstrip for right removal of blank spaces and .strip for clear all the blank spaces present in the string
Use of suffix and prefix
If you want to use some prefix you can do it with the use of just operator . I am telling you how to do it. It is quite simple by looking at this you will know how to do this.
Thursday, 23 March 2017
Ruby Programming Language Tutorials Lec 11
Tutorial 11 : Strings
Multi line String
We make multi line string very easily. Just by typing "multiline_string" and it is end with = and two less than sign and after that type in capital EOM. Now your multiine string is start and inside it you can write very long string and in this we can do interpolation also like we can write # {4 + 5} and also give new line command by using \n. Let's do practically what I said to you. Check it from the below diagrams
Input Output
Manipulation with strings
Now I will tell you about the several type of string printing. Such as addition of 2 strings by + symbol and by interpolation and also learn about a new type of keyword include by which we can search a string into another string. This language is also very good for string purposes.
In this we are making a variable name First_name and give it a value Derek
and make another variable name last_name and give the value Banas.
In third line we make another variable name full_name and give them the value first_name + last_name. After that make another variable of name middle_name and its value Justin. Than we add all three strings into one string name full_name. At last we search Justin into full_string. and than it will give the result true
For taking the size of the string just write string_name.size and it will return the size of the string you can print it with the help of print or puts.
for the count of vowels we is to simply write the string_name,count("aeiou") it will return the count of the vowels present in the string .
For the count of consonants we is to simply write string_name.count("^aeiou"). It will return the count of the consonants present in the string.
For the string string start with in the "Banas" Just type string_name.start_with?("Banas")
will return in true or false.
For the indexing of the string just write string_name.index
for the count of vowels we is to simply write the string_name,count("aeiou") it will return the count of the vowels present in the string .
For the count of consonants we is to simply write string_name.count("^aeiou"). It will return the count of the consonants present in the string.
For the string string start with in the "Banas" Just type string_name.start_with?("Banas")
For the indexing of the string just write string_name.index
Input Output
Tuesday, 21 March 2017
Ruby Programming Language Tutorials Lec 10
Tutorial 10 : Exceptions
Make your exceptions
Here we are creating our new variable name age
whose value is give 12 and then we define a function of name “change_age” in
which we passing the value of age raise argument error the value of age is
greater than zero than until it will print “Enter Positive number” Then we will
end the function.
And then we create a loop which will take the values of the age. If it’s negative value it will go under the argument error will print “That is an impossible age”. And then we end the loop of the begin then program will execute and givers the result “That is an impossible age”.
Input Output
Difference between double quotes and single quote in the string function in puts
Alright
let’s start by typing an line of the puts in First one we write in double
quotes write “Add Them #{4+5} \n \n” .
and in the Second line we will write puts ‘Add Them #{4+5} \n \n’. and the execute it so lets see what
will happen.
Input Output
Monday, 20 March 2017
Ruby Programming Language Tutorials Lec 09
Tutorial 9 : Functions
Definition of the functions
To define functions we is to type def to define function and the give a space and write the name of the functions then in braces pass the parameters if any. If no so give only open and close braces and then if you want to return the value or not. If you want to return a value so type return and then type to which is to be return if it is string type .to_s and if it is integer so type .to_i
For example of a function of addition of two numbers we can write it like this
Input Output
In this the function is called by value 3 and 4 by which it return and give the output 7
Change the value under a function
This part of tutorial is to understand that when the value of an integer get changes and when it takes new values. So check this program and try to understand.
Input Output
In first line we give the value of x to 1 and after that we create function of change_x and pass the value of x and third line and we change thw value of x from 1 to 4. and in 5th line we end the function and in 6th line we call the function by the name and in 7th line we print the value of x
Any value change inside the function will remain in the function it will not affect the outside. It is so important when you work on the functions.
Exceptions
We can catch exception by both catch and rescue
Input Output
In this program we are catching the exceptions by rescue function here we are saying that rescue all the conditions when user want to divide his number by zero.
Subscribe to:
Posts (Atom)
-
Tutorial 1 Starting with Ruby Programming language Hello every one welcome to my ruby programming tutorials. In this I am gonna...
-
Tutorial 8 : Cont. Looping For Loop For the for loop creation I am not going to take your much time. watch in the picture and i a...
-
Tutorial 4 Every thing is a class in ruby In ruby every thing is a class to see which thing is in which class just type puts fo...